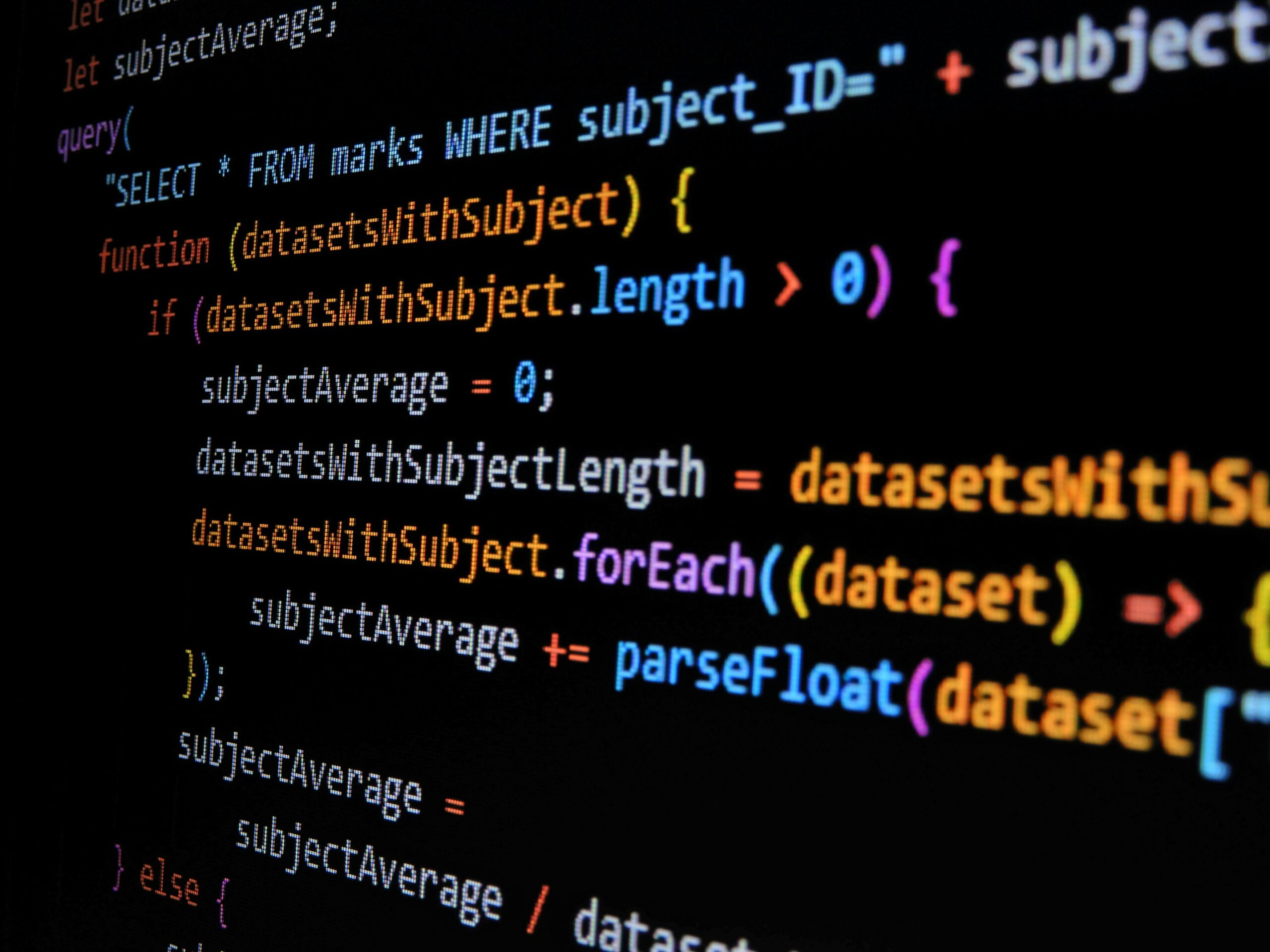
Let’s Learn Javascript. Embark on an exciting journey into the world of web development as we unravel the mysteries of JavaScript, the backbone of interactive and dynamic web experiences.
Let’s start with some questions
Where does JavaScript code run?
Originally designed to run exclusively in browsers, JavaScript has undergone a transformation with the advent of Node.js. Created by Ryan Dahl in 2009, Node.js allows JavaScript code to run outside the browser environment. This means developers can use JavaScript to build the backend for web and mobile applications. Each browser has its JavaScript engine, like SpiderMonkey in Firefox and V8 in Chrome. Node.js incorporates Google’s V8 JavaScript engine into a C++ program, providing a runtime environment for executing JavaScript code outside the browser. In summary, JavaScript code can run both in a browser and in Node.js, broadening its application possibilities
What is the difference between JavaScript and ECMAScript?
ECMAScript (ES) is not a language itself; instead, it serves as a specification. JavaScript is a programming language that adheres to the ECMAScript specification. The ECMAScript specification is maintained by Ecma International, defining standards for JavaScript. The first version of ECMAScript was released in 1997, and annual releases have occurred since 2015. ECMAScript 2015, also known as ES6, brought numerous new features to JavaScript. It’s crucial to understand that JavaScript is the practical implementation of the ECMAScript specification, ensuring consistency and compatibility across different platforms.
Variables
In traditional JavaScript (pre-ES6), the var
keyword was used to declare variables. However, with the introduction of ES6, the let
keyword is now the recommended practice for variable declarations. For instance:
let name;
Constants
While variables allow us to store and manipulate data, there are instances in real-world applications where we want to ensure that the value remains constant throughout the program’s execution.
Let’s consider an example where we declare a variable named interestRate
and initially set it to 0.3:
let interestRate = 0.3;
interestRate = 1;
console.log(interestRate);
To declare a constant, you use the const
keyword. Let’s modify our example accordingly:
const interestRate = 0.3;
interestRate = 1;
TypeError: Assignment to constant variable.
In practice, the best practice is to default to using constants unless you explicitly need to reassign a variable. Constants provide stability to your codebase by ensuring that certain values remain constant throughout the execution of your program.
Variables/Constants
In JavaScript, the world of data is categorized into two main types: primitives, also known as value types, and reference types.
1. Strings
Strings represent sequences of characters and are declared using what we call a “string literal.” Here’s an example:
let name = 'Your String';
Strings are versatile and widely used for representing text and characters in JavaScript.
2. Numbers
Numbers are used to represent numerical values. They can be integers or floating-point numbers. For instance:
let age = 30;
In this example, the variable age
is assigned the number 30
.
3. Booleans
Booleans are logical values that can be either true
or false
. They are particularly useful in situations where we need to make decisions based on the truth or falsity of a condition:
let isApproved = true;
Here, isApproved
is a boolean variable set to true
.
4. Undefined
When a variable is declared but not initialized, its value is undefined
. For instance:
let firstName;
In this example, firstName
is declared but not assigned a value, making its default state undefined
.
5. Null
The null
value is used in situations where we intentionally want to clear the value of a variable. It is commonly employed when dealing with user selections. For example:
let selectedColor = null;
In this case, selectedColor
is initially set to null
. Later, if a user selects a color, we might reassign the variable to the chosen color. If the user wants to remove the selection, setting it back to null
serves that purpose.
6. Symbol
It represents a unique identifier that can be used as an object property. Unlike strings or numbers, symbols are guaranteed to be unique, even if they have the same name.
Creating Symbols: You can create a symbol using the Symbol()
function, like this:
const mySymbol = Symbol();
Optionally, you can pass a description as an argument to provide a human-readable description of the symbol:
const mySymbol = Symbol('mySymbolDescription');
Dynamic Typing in JavaScript
One of the distinguishing features of JavaScript that sets it apart from many other programming languages is its dynamic nature. JavaScript is classified as a dynamic language, offering flexibility when it comes to variable types. In contrast to static languages where the type of a variable is fixed at declaration and cannot change, JavaScript allows the type of a variable to evolve during runtime.
Let’s revisit the example of the name
variable from the previous lecture. In JavaScript, we can use the typeof
operator to inspect the type of a variable. Initially, name
is declared as a string:
let name = 'Your String';
console.log(typeof name); // Outputs: string
However, in the dynamic world of JavaScript, the type of a variable can change. If we later reassign name
to a number, the type dynamically adjusts:
name = 42;
console.log(typeof name); // Outputs: number
This ability to adapt the type of a variable at runtime is a characteristic of dynamic languages like JavaScript.
Exploring typeof
Operator:
Let’s examine a few more examples using the typeof
operator:
let age = 30;
console.log(typeof age); // Outputs: number
let isApproved = true;
console.log(typeof isApproved); // Outputs: boolean
let firstName;
console.log(typeof firstName); // Outputs: undefined
let selectedColor = null;
console.log(typeof selectedColor); // Outputs: object
An interesting observation is the type of firstName
being reported as undefined. This seemingly curious behavior arises from the fact that undefined
is considered both a type and a value in the primitive types category of JavaScript.
Objects
What is an Object?
An object in JavaScript, much like objects in real life, consists of properties that define its characteristics. Think of a person with attributes like name, age, and address. In JavaScript, when dealing with multiple related variables, these variables can be encapsulated within an object.
Creating an Object:
Let’s dive into the syntax of creating an object using an object literal:
let person = {
name: 'Marsh',
age: 30
};
In this example, person
is an object with two properties: name
and age
. The keys (name
and age
) are the properties, and the corresponding values ('Marsh'
and 30
) are the data associated with those properties.
Accessing Object Properties:
There are two primary ways to access object properties:
Dot Notation
console.log(person.name); // Outputs: Marsh
Bracket Notation
console.log(person['name']); // Outputs: Marsh
- Bracket notation is particularly useful when the property name is dynamic and determined at runtime.
Modifying Object Properties:
Properties of an object can be modified using either notation:
person.name = 'John';
console.log(person.name); // Outputs: John
Dot Notation vs. Bracket Notation:
While dot notation is concise and often preferred, bracket notation has its use cases, especially when property names are dynamic or not known in advance. For example:
let selection = 'name';
console.log(person[selection]); // Outputs: John
Choosing Between Notations:
- Dot Notation: Cleaner and preferable for most cases.
object.property
- Bracket Notation: Useful when dealing with dynamic property names.
object['property']
Arrays in JavaScript
Creating an Array:Let’s start by creating an array using the array literal, denoted by square brackets:
let selectedColors = []; // Empty Array
In this example, selectedColors
is initialized as an empty array.
Adding Elements to the Array:
You can add elements to an array using the array’s indices:
selectedColors[0] = 'red';
selectedColors[1] = 'blue';
Arrays in JavaScript are zero-indexed, meaning the first element is at index 0, the second at index 1, and so forth.
Displaying Array Elements:
To display elements from an array, you can use the console.log
statement along with the index:
console.log(selectedColors[0]); // Outputs: red
Dynamic Nature of Arrays:
JavaScript’s dynamic nature extends to arrays as well. You can dynamically modify the length of an array and mix different data types within it:
selectedColors[2] = 'green'; // Adding another color
selectedColors[3] = 42; // Adding a number
Now, selectedColors
contains three strings and one number.
Arrays as Objects:
Technically, arrays are objects in JavaScript. They inherit properties, and one such property is the length
property, which gives the number of elements in the array:
console.log(selectedColors.length); // Outputs: 4
Functions
Functions in JavaScript are the bedrock of building modular and reusable code. They encapsulate a set of statements, enabling tasks to be performed or values to be calculated. Let’s unravel the essence of functions with a few practical examples.
Function Declaration:
To declare a function, we use the function
keyword, followed by the function name and parentheses:
function greet(name) {
console.log("Hello " + name);
}
Here, greet
is the function name, and it takes one parameter name
. The logic of the function, enclosed in curly braces, is to log a greeting message to the console.
Calling a Function:
To invoke a function, we use its name followed by parentheses:
greet("John");
greet("Mary");
These function calls with different arguments result in personalized greetings on the console.
Function Parameters and Arguments:
Understanding the difference between parameters and arguments is crucial. Parameters are the variables declared in the function, like name
in our example. Arguments, on the other hand, are the actual values passed to those parameters during function calls. In our case, “John” and “Mary” are arguments.
Functions with Multiple Parameters:
Functions can have multiple parameters, separated by commas:
function greetWithLastName(firstName, lastName) {
console.log("Hello " + firstName + " " + lastName);
}
Here, greetWithLastName
takes two parameters, firstName
and lastName
.
Default Values:
If an argument isn’t provided for a parameter, JavaScript defaults it to undefined
. To avoid this, you can assign default values:
function greetWithDefaultLastName(firstName, lastName = "Doe") {
console.log("Hello " + firstName + " " + lastName);
}
Now, if no lastName
is provided, it defaults to “Doe.”
Multiple Function Calls:
Functions can be called multiple times with different arguments:
greetWithLastName("John", "Smith");
greetWithDefaultLastName("Alice");
This results in varying personalized greetings based on the provided arguments.